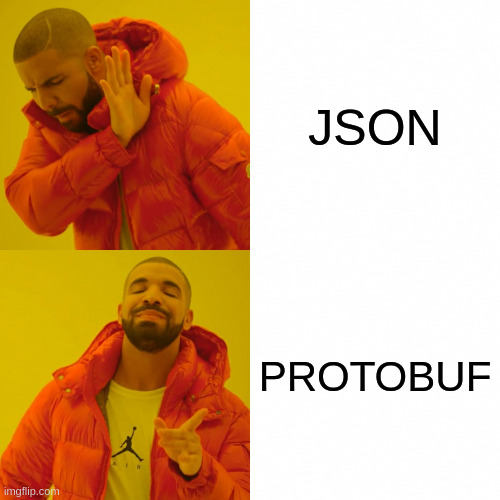
Py-x-Protobuf - Or How I Learned to Stop Worrying and Love Protocol Buffers
TLDR Protobufs are a mainstay of microservice development. You can use them in lieu of JSONs when interacting with a webservice. They’re much faster than JSON when you’re deserializing or serializing them. They’re designed mostly for applications that talk to each other. They’d make excellent choices for MCP-centric applications as well. Introduction I first heard about protobufs from a friend working at Gojek in 2017. I didn’t know what they were used for and even when I looked them up, I didn’t understand what I needed them for....